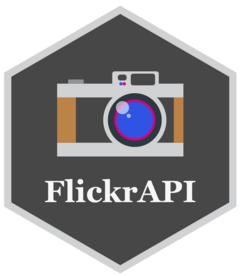
Search for photos on Flickr by user id, tags, license, or bounding box
getPhotoSearch.Rd
Use the Flickr Search API to return pages of photos sorted by date posted, date taken, interestingness, and relevance. Optional search parameters including spatial bounding box, user id, tags, and image license.
Usage
getPhotoSearch(
api_key = NULL,
user_id = NULL,
tags = NULL,
license_id = NULL,
sort = "date-posted",
desc = FALSE,
bbox = NULL,
img_size = NULL,
date = NULL,
upload_date = NULL,
extras = NULL,
per_page = 100,
page = NULL,
...
)
get_photo_search(
api_key = NULL,
user_id = NULL,
tags = NULL,
license_id = NULL,
sort = "date-posted",
desc = FALSE,
bbox = NULL,
img_size = NULL,
date = NULL,
upload_date = NULL,
extras = NULL,
per_page = 100,
page = NULL,
...
)
Arguments
- api_key
Flickr API key. If api_key is
NULL
, the function usesgetFlickrAPIKey()
to use the environment variable "FLICKR_API_KEY" as the key.- user_id
The NSID of the user with photos to search. If this parameter is
NULL
passed then all public photos will be searched.- tags
A vector of tags to search for.
- license_id
The license id for photos. For possible values, see the Flickr API method flickr.photos.licenses.getInfo or see details for more information. If license_id is provided, "license" is added to extras.
- sort
Order to sort returned photos. The possible values are: "date-posted-asc", "date-posted-desc", "date-taken-asc", "date-taken-desc", "interestingness-desc", "interestingness-asc", and "relevance" The trailing "-asc" or "-desc" indicator for sort direction is optional when using the desc parameter.
- desc
If
TRUE
, sort in descending order by the selected sort variable; defaults toFALSE
.- bbox
A object of class
bbox
or a numeric vector with values for xmin, ymin, xmax and ymax representing the bottom-left corner of the box and the top-right corner.- img_size
A character string with the abbreviation for one or more image sizes ("sq", "t", "s", "q", "m", "n", "z", "c", "l", or "o"). If a single img_size is provided the url, width, and height columns are renamed (e.g. img_url instead of url_sq) and an img_asp column is added to the results; defaults to
NULL
.- date
Date taken in any format supported by
as.POSIXlt()
. If date is a length 1 vector, it is treated as a minimum date taken with the maximum set one day later. If date has a length greater than 1, the minimum and maximum date from the vector are used. If date is provided, "date_taken" is added to extras.- upload_date
Date uploaded in any format supported by
as.POSIXlt()
. If upload_date is a length 1 vector, it is treated as a minimum date uploaded with the maximum set one day later. If date has a length greater than 1, the minimum and maximum date from the vector are used. If date is provided, "date_upload" is added to extras.- extras
A vector of extra information to fetch for each returned record. Currently supported fields are: c("description", "license", "date_upload", "date_taken", "owner_name", "icon_server", "original_format", "last_update", "geo", "tags", "machine_tags", "o_dims", "views", "media", "path_alias", "url_sq", "url_t", "url_s", "url_q", "url_m", "url_n", "url_z", "url_c", "url_l", "url_o")
- per_page
Number specifying how many results per page to return. Default 100 results per page. Maximum of 250 if
bbox
provided or 500 otherwise.- page
Number specifying which search results page to return. Default is page 1 of results returned.
- ...
Dots can be used to pass any additional arguments supported by the flickr.photos.search API method excluding license, min_taken_date, max_taken_date, min_upload_date, and max_upload_date which are supported by other named parameters. Dots also support two legacy parameters: licence_id (variant spelling for license_id) and geo (set
geo = TRUE
to include "geo" in extras).
Details
License id options:
license_id can be an integer from 0 to 10 or a corresponding license code including:
"c" (All Rights Reserved),
"by-bc-sa" (Attribution-NonCommercial-ShareAlike),
"by-nc" (Attribution-NonCommercial),
"by-nc-nd" (Attribution-NonCommercial-NoDerivs),
"by" (Attribution),
"by-sa" (Attribution-ShareAlike),
"by-nd" (Attribution-NoDerivs),
"nkc" (No known copyright restrictions),
"pd-us" (United States Government Work),
"cc0" (Public Domain Dedication),
or "pd" (Public Domain Mark).
See also
Flickr API Documentation: flickr.photos.search
Examples
if (FALSE) {
# Search for photos tagged "cats" and "dogs"
# Return images in descending order of date taken
getPhotoSearch(
api_key = get_flickr_api_key(),
sort = "date-taken-desc",
tags = c("cats", "dogs")
)
}
if (FALSE) {
# Search for photos uploaded to the NPS Grand Canyon user account.
# Return extra fields including the date taken and square image URL.
getPhotoSearch(
api_key = get_flickr_api_key(),
user_id = "grand_canyon_nps",
extras = c("date_taken", "url_sq")
)
}
if (FALSE) {
# Search for photos tagged "panda" in the area of Ueno Zoo, Tokyo, Japan
getPhotoSearch(
api_key = get_flickr_api_key(),
tags = "panda",
bbox = c(139.7682226529, 35.712627977, 139.7724605432, 35.7181464141),
extras = c("geo", "owner_name", "tags")
)
}